Counting Bits
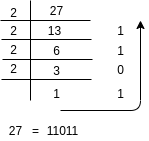
Given a non negative integer number num . For every numbers i in the range 0 ≤ i ≤ num calculate the number of 1's in their binary representation and return them as an array. Input: 2 Output: [0,1,1] Follow up: It is very easy to come up with a solution with run time O(n*sizeof(integer)) . But can you do it in linear time O(n) /possibly in a single pass? Space complexity should be O(n) . Can you do it like a boss? Do it without using any builtin function like __builtin_popcount in c++ or in any other language. Now, It is time to think before we write our code onto a paper. We read about the binary representation in college. A binary number is a special type of representation of a number in a binary format where the bit can be 0 or 1. You had an idea that the computer system also understands the 0's and 1's. 0's mean an inactive bit and 1's mean an active bit. Let's understand the easiest way to calculate the number using the pictorial dia